Class and Object in Kotlin
Welcome back to our introductory series on Kotlin. In this article, we’ll start to dig into how Object-Oriented Programming (OOP) works in Kotlin. We’ll cover class and object and, of course, give you some code examples that you can learn from.
According to the principles of OOP, classes are a kind of blueprint of a runtime entity and objects are their state, which include both behavior and state. In Kotlin, class declarations are comprised of a class header and a class body, surrounded by braces. Sound familiar? It’s just like in Java! Have a look:
Class myClass { // class Header
// class Body
}
And just like in Java, Kotlin also lets you create multiple objects of a class. The programmer is free to include its class members and functions. We can control the visibility of a class member’s variables using various keywords which we’ll talk about in a future article on Visibility Control. Let’s look at an example where we create one class and its object which we’ll use to access different data members of that class:
class myClass {
// property (data member)
private var name: String = "Discover.SDK"
// member function
fun printMe() {
print("Get all the development tools you need at: "+name)
}
}
fun main(args: Array<String>) {
val obj = myClass() // create obj object of myClass class
obj.printMe()
}
This bit of code will return the following output in the browser, where we are calling printMe() of myClass using its own object.
Get all the development tools you need at: Discover.SDK
Nested Classes
When a class has been created inside of another class, we call this a nested class. Nested classes in Kotlin are static by default, so they can be accessed without creating an object of that class. Here’s an example in which we demonstrate how Kotlin interprets a nested class.
fun main(args: Array<String>) {
val demo = Outer.Nested().foo() // calling nested class method
print(demo)
}
class Outer {
class Nested {
fun foo() = "Welcome to our Kotlin series!"
}
}
Here is the output from this code:
Welcome to our Kotlin series!
Inner Classes
When a nested class is designated as “inner” then we call it (no surprise) an Inner class. Inner classes are accessed by the data member of the outer class. Here’s an inner class example where we access the data member of the outer class.
fun main(args: Array<String>) {
val demo = Outer().Nested().foo() // calling nested class method
print(demo)
}
class Outer {
private val welcomeMessage: String = "Welcome to the DiscoverSDK’s Kotlin Series"
inner class Nested {
fun foo() = welcomeMessage
}
}
This code will give you the following output:
Welcome to the DiscoverSDK’s Kotlin Series
Here we’re calling the nested class using the default constructor that the Kotlin compiler provides at compile.
Anonymous Inner Classes
Anonymous inner classes are a pretty handy feature that makes the life of a programmer a lot easier. Whenever we implement an interface, a concept known as an anonymous inner block comes into play. The concept of creating an object of the interface using a runtime object reference is what’s known as an anonymous class. In this example, we’ll create a quick interface and then create an object of that interface using the anonymous inner class mechanism.
fun main(args: Array<String>) {
var programmer :Human = object:Human // This creates an instance of the interface
{
override fun think() { // This overrides the think method
print("I am an Anonymous Inner Class example")
}
}
programmer.think()
}
interface Human {
fun think()
}
This code will return the following output:
I am an Anonymous Inner Class example
Type Aliases
Type aliases are a property of the Kotlin compiler. They provide the flexibility of creating a new name of an existing type, but don’t actually create a new type. If the type name is too long, you can easily give it a shorter name which you can use in the future. Type aliases are quite handy for complex types. In the newer version of Kotlin, they removed support for type aliases. But if you’re using an old version you may still want to use it. If so, it goes a bit like this:
typealias NodeSet = Set<Network.Node>
typealias FileTable<K> = MutableMap<K, MutableList<File>>
That’s all for this article. Check back soon for the next article in the series where we’ll be taking a closer look at constructor in Kotlin. See you then!
Previous Article: Control Flow in Kotlin | Next Article: Constructors & Inheritance in Kotlin |
Recent Stories
Top DiscoverSDK Experts
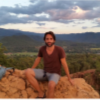
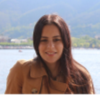
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment