Beginners' introductory guide to web development with Python and Django
Django is the most popular and well architected full stack web development framework in the realm of Python. If you love Python and you want to work with the web then it’s Django to the rescue. There are a lot of Python web frameworks and micro frameworks but none of them is as well architected as Django. Everything in Django is written in pure Python code. The configuration file (settings.py) is also written in Django. You don’t need to write a single line of SQL for talking to a database, you don’t need to create the schema, and you don’t need to worry much about what database system is backing your persistent layer. Database things (models) in Django aire written in pure Python. So, you don’t need to know any other alien language to work with Django. You get a full stack web framework out of the box.
Why Django
If Python is your primary programming language and you want to work with the web then there is nothing better and more professional than Django. If you want everything in one place ranging from templating to ORM then Django is the best in Python. Above all if you want a better architecture you need Django. Again, if you want to scale your business and never be lost in a stack of badly written code then you need Django. Complete documentation might be another reason for choosing it along with a large, active community spread all over the web.
Installing and creating a virtual environment
You can use Django without using virtualenv. But it is always good practice to use virtualenv not only for Django projects but also for all types of python projects unless it’s a project without any external library dependency. Again, if you need multiple versions of Python for a variety of requirements then you need it all the time. Above all when you need a separate Python environment regardless of anything you must go for virtualenv. In different virtualenv you can use different versions of the same library e.g. we can use multiple version of Django. There is no limitation on how many virtualenv a system hosts.
Install virtualenv with pip
pip install virtualenv
Create a virtualenv
virtualenv venv
A python virtual environment will be created with the name venv in the current working directory.
Activate the virtual environment on Unix/Linux (assuming that your virtualenv name is venv)
$ source venv/bin/activate
On Windows powered machines
venv\Scripts\activate
Deactivate the active virtualenv
deactivate
Installing Django
No matter whether you are on a virtualenv activated shell or not, the Django installation command is the same.
pip install django
This will install the latest version of Django. At the time of writing this article the latest stable version of Django is 11.1.3. You may see a different version when you are working. Try to use the latest version of Django and try not to use any lower version of Python than Python 3.5. Soon Python 2.x is going to live in a museum and Django is dropping support for all lower versions of Python from Django 2.0.
Creating your first Django project
Before you can start creating Django apps you need to create a Django project. With a single line of command your initial Django project will be ready with handsome default settings and some useful generated code. Let's call our project by the name of discover_project. I like and recommend to append _project at the end of the project name as it will save you from a lot of hassles including the confusion with Django app names.
django-admin startproject discover_project
Now, you will see a directory is created in the current working directory with another directory inside with the same name. The inner discover_project directory is the original project name and you must not rename this to anything else (it is possible to rename it but then you have to change some configuration). The outer discover_project is just a name and you can change it to whatever you like. Inside the outer discover_project you can see a file called manage.py which is the gateway to command Django to run management commands.
Running Django web applications
You need a web server to run a webapp. For Django you do not need to install another piece of software to run a server at the development stage. A default server comes with Django that is actually a simple python HTTP server. You do not need to write some configuration file to run it. With Django management command you can make your server go live within seconds. At first you need to change your directory to discover_project and then use the runserver management command.
cd discover_project
python manage.py runserver
A server will be started with the default port of 8000. You can change the default port if you like with the help of command line parameter. Now, go and point your browser to http://localhost:8000 and if nothing went wrong you should see a default page that congratulates you on your success like the following.
It worked!
Congratulations on your first Django-powered page.
Next, start your first app by running python manage.py startapp [app_label].
You're seeing this message because you have DEBUG = True in your Django settings file and you haven't configured any URLs. Get to work!
Creating your first Django app
In Django you can create multiple apps inside a single project with all the separation of router/urls, views, models, templates, static resources. To create an app change your directory to the project directory and run the following command. Let's say our first app is called app1
python manage.py startapp app1
Adding your app to the project
You cannot use the components of your app without adding it to the settings.py file. Open the settings.py in your favorite code editor or IDE and find the the array called INSTALLED_APPS. Now, place app1 as a string at the end of the array.
Now, the array should look like the following:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
# My apps
'app1'
]
Django URL dispatcher/Router and View
Before serving any type of resources from within a Django web application, the Django system needs to know what view it should call. But on the web almost nothing can exist without some kind of URL. The decision of calling view is taken by the router or the list of urls. You can find and set the urls inside the project folder's urls.py file's urlpatterns array. You can also include other urls from different apps.
Go to the project folder (inner discover_project) and open the urls.py file with your editor. The content of the file should look like the following:
from django.conf.urls import url
from django.contrib import admin
urlpatterns = [
url(r'^admin/', admin.site.urls),
]
The url() function is used for creating an url instance and all those instance objects should reside in the urlpatterns array. For now we do not need admin and you can safely comment that out or remove it. urlpatterns inside the project's urls.py is the primary point where routing happens. If you want to route from other apps you need to include them here. To include you need to use the function include().
Let's go to the app1's directory and create a file named urls.py and create some urls there inside urlpatterns with url(). The function urls() takes few arguments and most important, one of them is the pattern of the url as a regular expression and the second is the view function. We want to create a url that will be /hello and the view function would show Hello DiscoverSDK.
The content of discover_project/urls.py should look like the following:
from django.conf.urls import url, include
from app1 import urls
urlpatterns = [
url(r'^', include(urls))
]
The content of app1/urls.py should look like the following:
from django.conf.urls import url
from app1.views import hello
urlpatterns = [
url(r'^hello$', hello)
]
The content of app1/views.py should look like the following:
from django.http import HttpResponse
def hello(req):
return HttpResponse("Hello DiscoverSDK")
Fire up the built in Django server and go to http://localhost:8000/hello with your browser and you will see:
Hello DiscoverSDK
If you do not understand some parts of it then continue reading the following sections and read more articles in this series.
Django Views
In simple words Django view is a function or callable that returns a HttpResponse object or instance of any subclass of HttpResponse. Django has class based views but those views to be used by Django must be converted to some kind of callable before being used. Calling as_view() on class based views it can be converted to a callable and be usable with url().
We already have Django view in the previous section.
from django.http import HttpResponse
def hello(req):
return HttpResponse("Hello DiscoverSDK")
Our view function is called hello and it returns an object of HttpResponse class. A view is like a controller in other frameworks. The view will decide on business logics, retrieve data from database and a whole bunch of things. When a view needs to handle more than an HTTP GET request then it becomes complex to handle with a functional view. We can use a class based view in that case. In future articles I will cover them in detail.
Django Template
We cannot go far by just using a view that returns a hand coded string. We need to use some kind of templating system. In Django you do not need to go somewhere else or include some other third party engine for using template. Django has its own templating system.
Django has some sensible default as convention over configuration. You can put template files inside templates directory of app's root directory. We do not want to make some conflict in template resolution between apps. So, we are going to create a directory as app1/templates/app1. Create a file in there with the name hello-template.html and put the following content.
<!DOCTYPE html>
<html>
<body>
<h1>DiscoverSDK</h1>
<p>This text is from hello-template.html template.</p>
</body>
</html>
Now, create a function inside views with the name hello_template. Now our views.py looks like the following:
from django.http import HttpResponse
from django.shortcuts import render
def hello(req):
return HttpResponse("Hello DiscoverSDK")
def hello_template(request):
template = "app1/hello-template.html"
return render(request, template)
Open the app/urls.py` file and add the view with the urls. The urls file should look like the following:
Fire up the server again and go to http://localhost:800/hello-template and you should see the following output:
DiscoverSDK
This text is from hello-template.html template.
View the source of the page and you will see:
<!DOCTYPE html>
<html>
<body>
<h1>DiscoverSDK</h1>
<p>This text is from hello-template.html template.</p>
</body>
</html>
Django template is a broad topic and I will cover it in future articles.
Django Models
Models are used for the persistent layer of Django web apps. Models is where database things happen. Models are usually defined inside apps' models.py file. You declare model classes inheriting from django.db.models.Model class and define its fields. You can imagine the classes as database schema, class instances as rows and instance attributes as columns. Models are a large topic and I am going to write dedicated articles on them as well. Again I do not consider it necessary to work with models at the earliest stage of learning Django.
In future lessons I am going to go through every single component of Django in separate articles. In the meantime you must practice all the code I have shown here and clarify the concepts.
Recent Stories
Top DiscoverSDK Experts
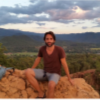
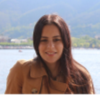
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment