Basics of JavaScript Functions
A function is a reusable block of code that you can use again and again by referring to its name. Again, you can make it act differently by passing different values to it during every use. Functions help us keep our code more modular and DRY (Do Not Repeat Yourself).
In this article I will help you understand different aspects of creating and using functions in JavaScript.
Getting Started
As mentioned in previous JavaScript related articles where I already discussed why I like to use Node.js for teaching and practicing JavaScript code, I am going to use it in this article too. So, you need to have the latest version of NodeJs installed on your system in order to follow along. Go to the official website of Node.js to see what instructions they provide for obtaining Node.js for your platform. As an IDE or code editor you can use whichever you like most. It will be better if it supports JavaScript syntax highlighting. You could even use a plain text editor for editing your code.
Create a file called have_fun.js in your preferred working directory. Keep it empty at this point. Point your command line to this directory and execute node have_fun.js to check if everything is alright.
Defining a Function
We can create, declare, or define a function with the function keyword. After the function keyword you have to type the function name, which must follow the naming conventions of JavaScript identifiers. In a generalized manner it can be said that they must not contain any characters other than letters, numbers, underscores and dollar signs. After the name is specified, you have to put parentheses. Inside the opening and closing parenthesis you can specify some parameters if you need. And then you have to put curly braces. The real code goes here. So, a simplified representation of a function should look like the following:
function functionName(){
// Our code goes here.
}
Let's put some real code inside the curly braces. For now, I just want to show a message on the console.
function functionName(){
console.log("Hey, you! Can you see me on the screen?");
}
Now, let's run the have_fun.js file by executing the node have_fun.js from the command line.
After executing the file, there is no text displayed on the screen. No error message is there either. That means that our code ran fine but it did not serve our purpose. It did not do anything because we did not call the function.
Calling/Invoking a Function
Unlike ordinary statements/instructions written inside a JavaScript file or script, the statements/instructions inside a function are not called until you explicitly call them. By calling a function we mean to run the block of code inside the function body (the block of code inside the curly braces). We use the words call, invoke or execute for talking about executing the function body. To call a function you need to put the name of the function followed by round braces. If you had specified any parameters inside the function definition, you can put specific values for each invocation of the function. So, we make that line go to the console by calling the function like below.
function functionName(){
console.log("Hey, you! Can you see me on the screen?");
}
functionName();
Now, execute the js file again to see the following output:
Hey, you! Can you see me on the screen?
As mentioned previously you can call or reuse a function as many times as you want:
function functionName(){
console.log("Hey, you! Can you see me on the screen?");
}
functionName();
functionName();
functionName();
functionName();
Execute the file to see the following output:
Hey, you! Can you see me on the screen?
Hey, you! Can you see me on the screen?
Hey, you! Can you see me on the screen?
Hey, you! Can you see me on the screen?
So, the same action was performed four times without repeating the lines of code inside the function body. It helped us to keep our code DRY and reusable.
Function Parameters
The body of the function in the above function is totally static. No matter how many times you call it, it does the same exact thing. We need it to be wiser and more dynamic. We want it to take decisions differently at different times. To do that we need to pass some extra data to the body of the code when we call or invoke it. To pass extra data to a function during invocation we need to declare some parameters when defining the function. Parameters are a set of variable names separated by commas inside the round braces.
function functionName(parameter1, parameter2, parameter3){
// Our code here
}
When we call the function we need to pass actual data as a variable or literal values in place of those parameters.
functionName("Value for parameter1", 2, "The value for the third parameter");
The parameters are available inside the function body as local variables. You are free to use them any way you like.
Let's define a function that will greet someone depending on their sex:
function salute(name, sex){
if (sex == "male"){
console.log("Hello Sir, how are you?");
}else if (sex == "female"){
console.log("Hello Mam, how are you?");
}else{
console.log("Hello, how are you?");
}
}
salute("Max", "male");
salute("Kathy", "female");
salute("Miix", "unknown");
Running the script shows the following output:
Hello Sir, how are you?
Hello Mam, how are you?
Hello, how are you?
Return Values
After calling a function we may be interested in getting some data from it. For example, we may need to know whether it could execute all the tasks properly that we told it to do. We can return this as an status text or status code. Returning value is as simple as putting the return keyword before a variable or literal values.
function doSomething(){
var status;
// Some useful code goes here
// Some useful code that sets the value of the status variable
return status;
}
Conclusions
Functions are like magic wands that help us to take different decisions in our code without repeating hundreds of lines. In this article I have just shown the basics of functions but there is a lot of other unexplored territory yet to cover. In future articles I would try to reflect on different parts of those territories. Functions in JavaScript have some dark sides too—more on the in the future.
Recent Stories
Top DiscoverSDK Experts
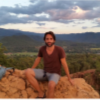
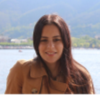
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment