Asynchronous Python Web Applications with aiohttp
Python is a land of wonder. It has many awesome features and a lot of convenient libraries for almost all of your needs. It has full stack web frameworks like Django, awesome and cute micro-framework like Flask, asynchronous servers like Tornado, and the infamous Twisted.
But among all those good things there was also a very bad one—Python was not asynchronous by default. Not only Python but many other language environment did not have that. JavaScript was asynchronous from the day one. And to serve web applications in an asynchronous way there is a great environment called NodeJs and a lot of framework built upon that.
It's not long that Python started its asynchronous journey keeping asynchronicity at its core. In the Python version 3.5 the asynchronous feature of Python became stable and something that we all started to not only like but love. Now we can use asyncio and the awesome keywords like async def, async with, await, etc.
But we still had one scarcity in the land of Python. We did have frameworks like Django and Flask but they did not support the asynchronous paradigm. And they still don't. aiohttp came as a savior. aiohttp is built upon Python asyncio and Python programmers no longer need to regret that we do not have any feature like NodeJs Python. We now have it and it has better performance than NodeJs. NodeJs is a great platform, but if you are Python programmer and do not want to switch from Python to JavaScript/NodeJs then aiohttp is the correct answer for you.
Before You Begin
This is not a beginner Python guide. This is a beginner aiohttp guides. So, it leads us to the following list to make sure that you are ready to rock and roll.
- Make sure that you have advanced knowledge in Python programming
- Make sure that you understand what is asyncio in Python and how to work with it
- Make sure that you know how to use async, await, etc. keywords
- You should have Python 3.5+ installed on your system
- You should have good familiarity with working with command lines on your system
Preparing Working Environment
Create or choose a directory where you want to keep the Python files we want to create and to create our virtual environment. Create a Python file called async_web.py in that directory.
You can edit Python code needed for this guide in any plain text editor. It's better to use a code editor with syntax highlighting or you can also use a Python supported IDE.
Creating a Virtual Environment
aiohttp is not a built-in Python library, it is an external one. In such situations when we need external libraries for our projects we should use a virtual environment to save our default Python environment from being messed up, using different libraries in different virtual environments and for some other reasons.
To work with virtual environment for Python you need to install virtualenv with the help of pip. Here's how we do it:
pip3 install virtualenv
Now open you command line in the chosen directory or cd into it. During the creation of the virtual environment you need to give it a name. A directory will be created with that name and all the necessary things for the current virtual environment will stay there. When you install any library with the help of pip in the activated directory, the library files will be saved there.
Create a virtual environment in the current directory or anywhere you like. I am going to name the virtual environment as aioenv, you are free to name it anything else.
virtualenv aioenv
Now you need to activate it. If you are on a UNIX/Linux based machine then you can run the following command:
$ source aioenv/bin/activate
If you are on a Windows machine you can run to following command for activating it.
aioenv\Scripts\activate
You will see (aioenv) on the left side of the prompt of your command line that indicates that you are inside a virtual environment.
You can deactivate it with the help of deactivate command:
deactivate
Now as we are in the virtual environment, we can install aiohttp library with the following command:
pip3 install aiohttp
Writing Your First Asynchronous Web Application
aiohttp can be used as both client and server. In our case we need the server part of it. Let's import aiohttp first.
from aiohttp import web
We need to create an application by instantiating the Application class from aiohttp.web.Application.
from aiohttp import web
app = web.Application()
Now, we need to define a function that will serve web requests from the users of our application. Let's call this function aio_home. The request serving functions will accept a request object. Remember that all request serving applications must be preceded with the async keyword to tell that the request will be served asynchronously. The function will have to return a Response object after processing. Request object can be found at aiohttp.web.Response
from aiohttp import web
app = web.Application()
async def aio_home(request):
text = "Welcome to my asynchronous home page developed with the help of aiohttp in Python"
return web.Response(text=text)
Now the question arises: how aiohttp will know what url it should sever by the aio_home function? We need to set route for that. Router object is available on the application object as router. To add route we will call add_get() on it. It will accept the url path we want to serve as the first parameter and the function identifier as the second parameter.
from aiohttp import web
app = web.Application()
async def aio_home(request):
text = "Welcome to my asynchronous home page developed with the help of aiohttp in Python"
return web.Response(text=text)
app.router.add_get('/', aio_home)
The last thing we need to do is to run the application. Do it like below:
web.run_app(app)
So, our final code looks like this:
from aiohttp import web
app = web.Application()
async def aio_home(request):
text = "Welcome to my asynchronous home page developed with the help of aiohttp in Python"
return web.Response(text=text)
app.router.add_get('/', aio_home)
web.run_app(app)
Now you can run the application by running the python file we created with the following command:
python async_web.py
Go to your web browser and browse to:
http://localhost:8080
What do you see? You see the following output if everything is alright:
Welcome to my asynchronous home page developed with the help of aiohttp in Python
Hurrah! Our asynchronous web application is up and running.
Conclusion
This was an introductory guide to aiohttp. In future I will write more on aiohttp. Check out my other articles, guides and tutorials on this blog.
Recent Stories
Top DiscoverSDK Experts
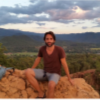
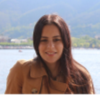
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment