AngularJS 1.x vs AngularJS 2 - Building Forms
When you’re building a website that deals with products that you are selling or a blog for gaining the exposure and audience for a specific niche, there are times when you’ll need to create a contact form on your page, which could be either the homepage or a separate "contact" page. Even though, HTML supports the <form> element that provides a basic support to collect user input (through several elements, such as, text fields, checkboxes, radio buttons, etc.), they are not sophisticated for most websites.
Thankfully, AngularJS supports highly sophisticated forms that provide data-binding and validation of input controls. It uses the help from several directives (i.e., ng-model, etc.) for data-binding which enables you to receive input from the user and even allows you to update the input simultaneously (basically a synchronize the model with view and view with a model!).
In this article, I will show you how to build forms in both AngularJS 1 and AngularJS 2 which will help you to decide which version to use for your next awesome website!
AngularJS 1 Form Example
In AngularJS, there is a directive called "ng-model" which provides the data-binding, it basically synchronizes the model to the view, which means the input from the JavaScript code will be binded to the rest of the application that you have written in HTML, meaning you can see the changes on the view the moment you start typing!
You provide the "ng-model" directive to the <input> element
<input type="text" ng-model="firstName">
Now, this property "firstName" can be referred in the "controller".
var app = angular.module('appname', []);
app.controller('mycontroller', function($scope) {
$scope.firstName = "Paul";
});
Forms use the component called controls, which basically contains three controls: input, select and textarea, which are the ways for a user to enter the data. In above we used the "input" control. The following is complete example of a simple form:
HTML
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<body>
<div ng-app="myapp" ng-controller="mycontroller">
<form>
First Name: <input type="text" ng-model="firstName">
</form>
</div>
<div>
First Name: {{firstName}}
</div>
<script>
var app = angular.module('myapp', []);
app.controller('mycontroller', function($scope) {
$scope.firstName = "Paul";
});
</script>
</body>
You can use JSFiddle (https://jsfiddle.net/) for validating/testing it.
If you want to synchronize the text input to the view, then you need to use "ng-app" in the <body> element and "ng-controller" in the <div> element. Also make sure you are printing the "firstName" in the <body> section somewhere. Following is an example of updated code:
HTML
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<body ng-app="myapp">
<div ng-controller="mycontroller">
<form>
First Name: <input type="text" ng-model="firstName">
</form>
<div>
First Name: {{firstName}}
</div>
</div>
<script>
var app = angular.module('myapp', []);
app.controller('mycontroller', function($scope) {
$scope.firstName = "Paul";
});
</script>
</body>
Creating checkboxes
Now, let's see how to create a checkbox. You need to use the "checkbox" in the input control. "checkbox" has two values: true and false. By default, it is set to false:
<input type="checkbox" ng-model="somevariable">
In order to use it in a practical way, we’re going to need to do something when a user clicks on the checkbox. We will use "ngShow" directive which hide/show the particular element. We will show the <h1> element when the users click on the checkbox.
HTML
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<body>
<div ng-app="">
<form>
Show the Name:
<input type="checkbox" ng-model="firstName">
</form>
<h1 ng-show="firstName">Paul</h1>
</div>
</body>
Creating radiobuttons
It is possible to use "radio" in the input control for the radiobuttons. What takes it apart from checkboxes is that it uses the "ng-model" directive which enables you to bind several radiobuttons that share the same "ng-model". For example, if there are several buttons that share the same property (i.e., does the similar task or belong to a same category), then you can apply the same "ng-model" to every buttons.
<input type="radio" ng-model="whichName" value="firstName">
<input type="radio" ng-model="whichName" value="secondName">
<input type="radio" ng-model="whichName" value="lastName">
Now, use these buttons effectively for a simple task. We’re to use "ng-switch" directive to swap the elements/expressions and "ng-switch-when" which works like IF-ELSE-THEN operations in other languages. The following is the complete code:
HTML
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<body ng-app="">
<form>
<input type="radio" ng-model="whichName" value="firstName"> First Name
<input type="radio" ng-model="whichName" value="secondName"> Second Name
<input type="radio" ng-model="whichName" value="lastName"> Last Name
</form>
<div ng-switch="whichName">
<div ng-switch-when="firstName">
<h1>First Name</h1>
<p>Richard</p>
</div>
<div ng-switch-when="secondName">
<h1>Second Name</h1>
<p>John</p>
</div>
<div ng-switch-when="lastName">
<h1>Last Name</h1>
<p>Paul</p>
</div>
</div>
</body>
Creating selectbox
Select boxes are like menus, meaning that it contains several options.
<select ng-model="whichName">
<option value="">
<option value="first">First Name
<option value="second">Second Name
<option value="last">Last Name
</select>
In AngularJS, we use the <select> element including several options that share the same "ng-model" value. Use the previous "ng-switch-when" to show/hide the element when the particular element in selectbox is selected. The following is the complete code:
HTML
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<body ng-app="">
<form>
<select ng-model="whichName">
<option value="">
<option value="first">First Name
<option value="second">Second Name
<option value="last">Last Name
</select>
</form>
<div ng-switch="whichName">
<div ng-switch-when="first">
<h1>First Name</h1>
<p>Richard</p>
</div>
<div ng-switch-when="second">
<h1>Second Name</h1>
<p>John</p>
</div>
<div ng-switch-when="last">
<h1>Last Name</h1>
<p>Paul</p>
</div>
</div>
</body>
Creating sophisticated forms
Now, we will combine the previous knowledge to create a highly sophisticated submit form. Focusing on non-validation form at this moment. First, create several input control for different text fields. Then create two buttons: Submit and Reset. We will also create two lists: Current and Original, so that you will be able to see how you can update the text simultaneously as well. Following is the complete code:
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<body>
<div ng-app="myApp" ng-controller="formCtrl">
<h1>Submit Form</h1>
<form novalidate>
First Name:<br>
<input type="text" ng-model="user.firstName"><br>
Last Name:<br>
<input type="text" ng-model="user.lastName">
<br>
Address:<br>
<input type="text" ng-model="user.address"><br>
Phone Number:<br>
<input type="text" ng-model="user.phonenumber"><br>
Website:<br>
<input type="text" ng-model="user.website"><br>
<br>
<button ng-click="submit()">Submit</button>
<button ng-click="reset()">RESET</button>
</form>
<p>Current = {{user}}</p>
<p>Original = {{master}}</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('formCtrl', function($scope) {
$scope.master = {firstName:"Paul", lastName:"Richard", address:"New York City", phonenumber: "00112", website:"http://contactform.com"};
$scope.myFunc = function () {
$scope.user = angular.copy($scope.master);
$scope.myTxt = "You clicked submit!";
$scope.reset();
}
$scope.master = {firstName:"Paul", lastName:"Richard", address:"New York City", phonenumber: "00112", website:"http://contactform.com"};
$scope.reset = function() {
$scope.user = angular.copy($scope.master);
};
$scope.reset();
});
</script>
</body>
You can learn more from here (https://docs.angularjs.org/guide/forms). Now, let's create a form with validation.
There are three validation flags: $dirty (it states that the value has been changed), $invalid (it states that value entered is invalid) and $error (it states the exact error). Following is the short code that demonstrates the validation:
<script src = "https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script> <body>
<h2>Form Validation Example</h2>
<div ng-app = "myapp" ng-controller = "mycontroller">
<form name = "studentForm" novalidate>
<table border = "0">
<tr>
<td>Enter first name:</td>
<td><input name = "firstname" type = "text" ng-model = "firstName" required>
<span style = "color:red" ng-show = "studentForm.firstname.$dirty && studentForm.firstname.$invalid">
<span ng-show = "studentForm.firstname.$error.required">First Name is required.</span>
</span>
</td>
</tr>
<tr>
<td>Enter last name: </td>
<td><input name = "lastname" type = "text" ng-model = "lastName" required>
<span style = "color:red" ng-show = "studentForm.lastname.$dirty && studentForm.lastname.$invalid">
<span ng-show = "studentForm.lastname.$error.required">Last Name is required.</span>
</span>
</td>
</tr>
<tr>
<td>Email: </td><td><input name = "email" type = "email" ng-model = "email" length = "100" required>
<span style = "color:red" ng-show = "studentForm.email.$dirty && studentForm.email.$invalid">
<span ng-show = "studentForm.email.$error.required">Email is required.</span>
<span ng-show = "studentForm.email.$error.email">Invalid email address.</span>
</span>
</td>
</tr>
<tr>
<td>
<button ng-click = "reset()">Reset</button>
</td>
<td>
<button ng-disabled = "studentForm.firstname.$dirty &&
studentForm.firstname.$invalid || studentForm.lastname.$dirty &&
studentForm.lastname.$invalid || studentForm.email.$dirty &&
studentForm.email.$invalid" ng-click="submit()">Submit</button>
</td>
</tr>
</table>
</form>
</div>
<script>
var mainApp = angular.module("myapp", []);
mainApp.controller('mycontroller', function($scope) {
$scope.reset = function(){
$scope.firstName = "John";
$scope.lastName = "Richard";
$scope.email = "contact@johnrichard.com";
}
$scope.reset();
});
</script>
</body>
You can read more here (https://docs.angularjs.org/api/ng/directive/form). The official documentation provides several examples in most directives and functions, so you can copy/paste several code to your form code to create your personal form of choice!
AngularJS 2 Form Example
Let's do a similar job in AngularJS 2. As you already know AngularJS 2 supports TypeScript, which allows you to write JavaScript code VERY quickly and easily with few lines of code. The end result is actually the JavaScript, because the transpiler converts it to JavaScript as that is the language that browser understands. Let’s start with it!
Use this (https://github.com/buckyroberts/angular-2-template) project as the start-up, because it has everything set-up. Download it and run the following comamnds in the directory:
$npm install
$npm start
It will open the web browser and you will see the "Angular 2 Template" written on a plain page. We are going to work with the files in "app/ts" directory. DO NOT TOUCH the "js" folder as it will contain the JavaScript files that are translated from TypeScript. So, open the "app.component" and write the following code (remove the previous code):
app.component.ts
import {Component} from 'angular2/core';
import {FormBuilder, Validators} from 'angular2/common';
@Component({
selector: 'my-app',
templateUrl: 'login-template.html'
})
export class AppComponent {
constructor(fb: FormBuilder) {
this.loginForm = fb.group({
email: ["", Validators.required],
password: ["", Validators.required]
});
}
doLogin(event) {
console.log(this.loginForm.value);
event.preventDefault();
}
}
Create a new file called "login-template.html" in the root directory, and copy the following code in it:
login-template.html
<form [ngFormModel]="loginForm" (submit)="doLogin($event)">
<input ngControl="email" type="email" placeholder="Your email">
<input ngControl="password" type="password" placeholder="Your password">
<button type="submit">Log in</button>
</form>
Now, if you save the files and run "npm start", you’ll see the simple login form. In this example, you can see that I have used the "ngModel" that simply binds the model to the view. We have created two inputs: email and password. The FormBuilder provides several components that automatically does several stuff, like validation, so if you type the email incorrectly (i.e., your '@' is missing), then it will mention it.
So, let's do something similar to previous example in AngularJS. We will create four fields this time: firstname, lastname, password and email. We will use the validation so that the users fill every field. Following is the complete code:
app.component.ts
import {Component} from 'angular2/core';
import {CORE_DIRECTIVES, NgClass, FORM_DIRECTIVES, Control, ControlGroup, FormBuilder, Validators} from 'angular2/common';
class User {
firstname:string;
lastname:string;
password:string;
email:string;
}
interface ValidationResult {
[key:string]:boolean;
}
class PasswordValidator {
static startsWithNumber(control: Control): ValidationResult {
if ( control.value && control.value.length > 0){
if (isNaN(control.value[0]))
return { 'startsWithNumber': true };
}
return null;
}
}
@Component({
selector: 'my-app',
templateUrl: 'login-template.html',
directives: [CORE_DIRECTIVES, FORM_DIRECTIVES]
})
export class AppComponent {
user:User;
studentForm: ControlGroup;
constructor(fb: FormBuilder){
this.user = new User();
this.studentForm = fb.group({
'firstname': new Control(this.user.firstname, Validators.required),
'lastname': new Control(this.user.lastname, Validators.required),
'password': new Control(this.user.password, Validators.compose([Validators.required,PasswordValidator.startsWithNumber])),
'email': new Control(this.user.email, Validators.required)
});
}
addNewGroup(user:User) {
if (this.studentForm.valid) {
alert('added ' + user.firstname + user.lastname + user.email);
this.user = new User();
}
else {
alert('Invalid Form! Please fill all the areas.');
}
}
}
login-template.html
<h2>Form Validation Example</h2>
<form [ngFormModel]="studentForm" class="form-horizontal" id='myForm' role="form">
<div class="col-md-7">
First Name: <input ngControl="firstname" #firstname="ngForm" [(ngModel)]="user.firstname" type="text" placeholder="firstname" class="form-control">
<span [hidden]="firstname.valid"><b>Required</b></span>
</div>
<div class="col-md-7">
Last Name: <input ngControl="lastname" #name="ngForm" [(ngModel)]="user.lastname" type="text" placeholder="lastname" class="form-control">
<span [hidden]="lastname.valid"><b>Required</b></span>
</div>
<div class="col-md-7">
Password: <input ngControl="password" #password="ngForm" [(ngModel)]="user.password" type="text" id="password" placeholder="password" class="form-control">
<span [hidden]="!password.control.hasError('required')"><b>Required</b></span>
<span [hidden]="!password.control.hasError('startsWithNumber')"><b>Must start with number</b></span>
</div>
<div class="col-md-7">
Email: <input ngControl="email" #email="ngForm" [(ngModel)]="user.email" type="text" id="email" placeholder="email" class="form-control">
<span [hidden]="!email.control.hasError('required')"><b>Required</b></span>
</div>
</form>
<br />
Valid: {{studentForm.valid }} <br />
<br />
<button type="button" (click)="addNewGroup(user)" class="btn btn-primary btn-lg" data-dismiss="modal">Submit</button>
The code is pretty much self-explanatory. We have binded the input controls with the AngularJS code, and we are checking if the input is valid from all the fields. You will get a nice login page when you do "npm start"! Try playing with it by adding new fields and validation logic. Read more about Forms here (https://angular.io/docs/ts/latest/guide/forms.html).
Conclusion
Over this past guide I have highlighted how to build forms in AngularJS 1 and AngularJS 2. You can see how dramatically AngularJS 2 changes the way you do web development. Even though many might argue that since AngularJS 2 isn't stable yet (as its new releases come frequently), so it is not good for stable development, it is nevertheless the right direction in a long-term.
If you have any questions, then please ask me in the comments section below!
Recent Stories
Top DiscoverSDK Experts
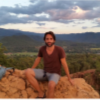
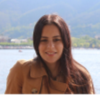
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment