Angular JS vs React by Example
It seems there have been countless posts written comparing these two popular JavaScript frameworks, and as always you can find opinions from both sides of the aisle. It appears that in most cases, a developer's opinion, which is often quite strong, is based on which library/framework/SDK etc. they've worked with the most. You work with it, you get used to it, you like it. Then you argue ferociously on the internet about it.
Thus it would appear that asking a question like “which is better— Angular or React?” is akin to asking which of the two happens to be more popular, which is not always the best gauge. So what about new developers who have to decide? The fact that one product is more popular than the other is not proof that it's better, and we can see examples of this with many products.
So in this post I won't be asking which is better, rather I’ll be comparing these two famous frameworks with examples and will let the reader choose which they prefer, because there is a significant difference in each of their respective "coding styles."
The Demo - Data Grid
I want to create a simple data grid and I want to be able to add, remove, and update the data inside of the grid, and also send all the grid's data as a big JSON object (for server handling).
This is how it looks like
Angular Approach - Divide and Conquer
AngularJS based on MVC design pattern. First we create the controller in our javascript code:
var app = angular.module("myApp", []);
app.controller("ResourceController", function($scope) {
$scope.resources = [{'ResourceAddress': 'http://www.discoversdk.com', 'ResourceDescription':'Great site'}];
$scope.RemoveResource = function (index) {
$scope.resources.splice(index, 1);
}
$scope.AddResource = function (newres) {
if ($scope.resources == null) {
$scope.resources = {};
$scope.resources = [];
}
$scope.resources.push(
{
ResourceAddress: newres.ResourceAddress,
ResourceDescription: newres.ResourceDescription,
});
newres.ResourceAddress = "";
newres.ResourceDescription = "";
}
$scope.ShowRes = function () {
alert(JSON.stringify($scope.resources));
}
});
Then the HTML file with the tags connected to the code:
<div ng-app="myApp" ng-controller="ResourceController">
<fieldset >
<h3>Add Resources</h3>
<span >Description:</span>
<input type="text" ng-model="newres.ResourceDescription" style="width:200px;" />
<span > URL:</span>
<input type="text" ng-model="newres.ResourceAddress" style="width:340px;" />
<a class="btn" href="#" ng-click="AddResource(newres)">Add</a><br/>
<h3>List of Resources</h3>
<table cellpadding="0" cellspacing="0">
<tbody>
<tr>
<th>Description</th>
<th>URL</th>
<th></th>
</tr>
<tr ng-repeat="res in resources">
<td><input style="width:200px;" type="text" ng-model="res.ResourceDescription" /></td>
<td><input style="width:440px;" type="text" ng-model="res.ResourceAddress" /></td>
<td><a class="btn" ng-click="RemoveResource($index)">Remove</a></td>
</tr>
</tbody>
</table>
<a class="btn" href="#" ng-click="ShowRes()">Show</a>
</fieldset>
</div>
And the CSS file for styles:
body
{
font-family:Arial;
color:#3a3a3a;
}
table
{
border:none;
text-align:left;
margin-bottom:20px;
}
tr th
{
background-color: #3b97d3;
color: #fff;
padding: 5px;
border-right: solid 1px #3b97d3;
border-left: solid 1px #fff;
}
tr th:first-child
{
border-left: solid 1px #3b97d3;
}
tr td
{
padding:5px;
padding: 5px;
border-right: solid 1px #d4d4d4;
}
tr td:first-child
{
border-left: solid 1px #d4d4d4;
}
table tr:last-child td
{
border-bottom: solid 1px #d4d4d4;
}
input[type="text"]
{
height:20px;
font-size:14px;
}
a.btn
{
color:#fff;
background-color:#3b97d3;
padding:0px 10px;
height:26px;
display:inline-block;
line-height:26px;
text-decoration:none;
border-radius:3px;
-moz-border-radius:3px;
-webkit-border-radius:3px;
cursor:pointer;
}
a.btn:hover
{
background-color:#73c7ff;
}
You can see and play with the code here
The React Approach - GUI with hierarchy
With reate we build the user interface from components with hierarchy and the main benefit is: code reuse
The main different is the mix of HTML, JavaScript and CSS styles but if we want to reuse the component in a new page we just need to copy one block
So in React we build a List Item for each row in our table:
var ResourceListItem = React.createClass({
getInitialState: function() {
return {ResourceDescription: this.props.children.ResourceDescription, ResourceAddress: this.props.children.ResourceAddress};
},
removeItem: function(e, index){
this.props.removeItem(resources.indexOf(this.props.children));
},
updateDescription : function(e){
resources[resources.indexOf(this.props.children)].ResourceDescription = e.target.value;
this.setState({ResourceDescription: e.target.value});
},
updateAddress : function(e){
resources[resources.indexOf(this.props.children)].ResourceAddress = e.target.value;
this.setState({ResourceAddress: e.target.value});
},
render: function(){
return (
<tr>
<td><input type="text" value={this.state.ResourceDescription} onChange={this.updateDescription} />{this.props.index}</td>
<td><input type="text" value={this.state.ResourceAddress} onChange={this.updateAddress} /></td>
<td><button onClick={this.removeItem.bind(null, this.props.children)}>remove</button></td>
</tr>
);
}
});
Then we build the table component:
var RecourceList = React.createClass({
render: function() {
var that = this;
var st = {
backgroundColor: '#3b97d3',
color: '#fff',
padding: '5px',
borderRight: 'solid 1px #3b97d3',
borderLeft: 'solid 1px #fff'
};
var st2 = {
padding: '5px',
borderRight: 'solid 1px #d4d4d4',
width: '250'
};
var createItem = function(itemText, index) {
return (
<ResourceListItem removeItem={that.props.removeItem} key={index}>{itemText}</ResourceListItem>
);
};
return (
<table>
<thead>
<tr style={st}>
<td style={st2}>Description</td>
<td style={st2}>Url</td>
<td></td>
</tr>
</thead>
<tbody>
{this.props.items.map(createItem)}
</tbody>
</table>
)
}
});
We then add the other grid components the same way and declare our application:
var MainApp = React.createClass({
getInitialState: function() {
return {items : resources};
},
addItems: function(newItem){
resources = resources.concat([newItem]);
this.setState({items: resources});
},
removeItem: function(itemIndex){
resources.splice(itemIndex, 1);
this.setState({items: resources});
},
show: function(){
alert(JSON.stringify(resources));
},
render: function(){
return (
<fieldset>
<div>
<h3>Add Resources</h3>
<AddResourceForm onFormSubmit={this.addItems} />
<a href="#" onClick={this.show}>Show</a>
<h3>List of Resources</h3>
<RecourceList items={this.state.items} removeItem={this.removeItem} />
</div>
</fieldset>
);
}
});
You can test and play with the code here (note that jsfiddle react support is a little limited so ti change the code you need to select regular javascript and then select back javascript 7 to run it).
Still can't choose between Angular and React? Then it's worth investigating into other JavaScript frameworks. We present the same practical example of Grid Control with 5 different frameworks including Knockout and Backbone.
Recent Stories
Top DiscoverSDK Experts
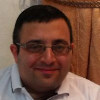
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment