An Introduction To the Go Programming Language
Go is an open source programming language designed for building simple, fast, and reliable software. The Go language was developed by Google Inc. and designed by Robert Griesemer, Rob Pike and Ken Thompson with the official announcement coming in November 2009. It now supports almost all major platforms like windows, Linux, OSx etc.
Features of Go:
- Omitting some OOP concepts is the one of the features of the Go Language—there won’t be any diamond inheritance issues, subclassing, or virtual methods to bother with.
- It is a statically typed language like C++ and java with excellent readability like python.
- It has all the basic data types with structure ‘Structs’ support.
- Though it is a statically typed language it has full support for unknown data.
- It has a faster compilation time than C.
- All the important data types are already implemented like maps, sets, queue etc.
- It supports networking and multithreading very well.
- Go is a great language for concurrent programs (programs with many independently running parts). Go provides many built in concurrency primitives like GoRoutines and channels.
- Remote package management is one of the great features of this language. All you have to do is go get <package_name> and your package is ready to use.
Note: the Go language supports the nil interface type which is a container for any type of data, you can assign any value to it and it will be stored to that interface.
Syntax
One of the best things about Go is its syntax. It is readable, easy to remember, and you don’t need to worry about semicolons.
The major blocks of the language like data type, decision making, loops etc. are traditional with some of them having a few minor tweaks.
Variable declaration is traditional as in C but in reversed as shown below:
int a;
is now
var a int
You can use one shorthand method to declare the variable and its type by
a := 5
It means you have declared a variable having the type integer with a value of 5.
Now the loops, go supports:
for, while, do-while, & range loops. Here are some examples:
for i := 1; i<=10: i++{
fmt.Println(2*i)
}
This code will print the table of 2.
some analysis of the code:
i:=1 will declare the variable i with a value of 1, its scope will be to the block itself only.
i++ will increase the value of i by one after one execution of the loop.
i<=10 is the condition for checking the value of i.
fmt.Println(2*i) here the fmt is the io package and Println is the function of this package which prints to the next line.
The for loop is very powerful here having so many other options, some of them are given below.
You can iterate over any array by using the range keyword with for
arr := []int{1,2,3,4,5}
for count, i := range arr{
fmt.Println(“array_value:”,i +,” counter: ”, count)
}
here:
arr is the array of int with given values
count is the counter variable which will be internally increased with every loop as you range over the array.
The “range” keyword is used to range over the given array
OUTPUT:
array_value: 1 counter: 1 array_value: 2 counter: 2 array_value: 3 counter: 3 array_value: 4 counter: 4 array_value: 5 counter: 5 |
Note: In Go you cannot declare a variable which is not used anywhere—this will give you a compile time error. Go also provides a feature to neglect the necessary declarations by using “_” in the place of that variable.
Now let’s talk about decision making. Go has if , if-else and switch statements which are used in the standard way.
Writing your First Go Program:
As mentioned before, Go offers readable, easy to remember syntax with no need to worry about semicolons, while the major blocks of the language like remain mostly traditional.
How to install go?
Linux:
$ sudo apt-get update
$sudo apt-get -y upgrade
$ wget https://storage.googleapis.com/golang/go1.7.4.linux-amd64.tar.gz
$ sudo tar -xvf go1.7.4.linux-amd64.tar.gz
$ sudo mv go /usr/local
Setup Go environment:
GOROOT is the location where you have installed your Go package.
$ export GOROOT=/usr/local/go
GOPATH is the location of your work directory. For example my project directory is
~/Projects/Proj1 .
$ export GOPATH=$HOME/Projects/Proj1
$ export PATH=$GOPATH/bin:$GOROOT/bin:$PATH
All the above environments will be set for your current session only. To make them permanent add the above commands to ~/.profile file.
Verify installation
$ go version
Note: For other platforms you can follow the link https://golang.org/dl/
helloWorld.go
package main
import(
“fmt”
)
func main(){
fmt.Println(“Hello World”)
}
run program:
$ go run helloWorld.go
Here we have named our file as a part of package “main”, which imports the package “fmt.” Here we are using the function Println() of the package fmt.
Go has a convention for using the functions and variables from any package i.e. if you want to make anything accessible outside of the package it should start with a capital letter. Go will internally follow that convention for checking the visibility of that variable or function. The dot operator is used for accessing the elements of the package.
Functions can return multiple values:
Functions in this language have a new feature which I think is one of the coolest. That is “functions can return more than one value.” Isn’t that awesome! An example is given below:
package main
func swap(a int, b int) (int, int){
a, b = b, a
return a, b
}
Now this is also magic: a, b = b, a this swaps a with b—that’s it.
Package system
Packages are also handled very beautifully. To use any package you just have to put the path of the package into the “import” block.
For making any package you just have to add the desired name of the package to the first line of the file i.e. package <name>.
Note: Package name must match the directory name.
Package main
import(
“fmt”
)
func main(){
arr := “ricky”
fmt.Println(“length of string”,”arr”,”is ”.len(arr))
}
Concurrency
Go implements concurrency with the help of goroutines and channels.
Goroutines
A goroutine is a function that is capable of running concurrently with other functions. To create a goroutine we use the keyword “go” followed by a function invocation:
Package main
import “fmt”
func hello(){
fmt.Println(“hello”)
}
func main(){
hello()
go hello()
}
This program consists of two goroutines. The first goroutine is implicit and is the “main” function itself. The second goroutine is created when we call “go hello()”.
Channels are the medium for two goroutines to communicate with one another and synchronize their execution. Channels implement internal logic for maintaining the concurrency between routines.
Final Note
Go is an emerging language due in part to its efficient compile time performance. Go compiles at supersonic speeds giving this language a definite “dynamic” feel. It is an absolutely minimalist language with only a handful of programming concepts and its utter simplicity makes it extremely easy to learn and to master. This is critical for practical software engineering. For further reading visit the official website: https://golang.org/
Visit the homepage - DiscoverSDK.com - tool for developers
Recent Stories
Top DiscoverSDK Experts
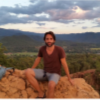
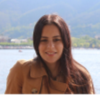
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment